# sphinx_gallery_thumbnail_number = 2 import numpy as np import matplotlib.pyplot as plt N = 21 x = np.linspace(0, 10, 11) y = [3.9, 4.4, 10.8, 10.3, 11.2, 13.1, 14.1, 9.9, 13.9, 15.1, 12.5] # fit a linear curve an estimate its y-values and their error. a, b = np.polyfit(x, y, deg=1) y_est = a * x + b y_err = x.std() * np.sqrt(1/len(x) + (x - x.mean())**2 / np.sum((x - x.mean())**2)) fig, ax = plt.subplots() ax.plot(x, y_est, '-') ax.fill_between(x, y_est - y_err, y_est + y_err, alpha=0.2) ax.plot(x, y, 'o', color='tab:brown') plt.savefig('confidence.jpg') plt.show()

""" ============ MRI With EEG ============ Displays a set of subplots with an MRI image, its intensity histogram and some EEG traces. """ import numpy as np import matplotlib.pyplot as plt import matplotlib.cbook as cbook import matplotlib.cm as cm from matplotlib.collections import LineCollection from matplotlib.ticker import MultipleLocator fig = plt.figure("MRI_with_EEG") # Load the MRI data (256x256 16 bit integers) with cbook.get_sample_data('s1045.ima.gz') as dfile: im = np.frombuffer(dfile.read(), np.uint16).reshape((256, 256)) # Plot the MRI image ax0 = fig.add_subplot(2, 2, 1) ax0.imshow(im, cmap=cm.gray) ax0.axis('off') # Plot the histogram of MRI intensity ax1 = fig.add_subplot(2, 2, 2) im = np.ravel(im) im = im[np.nonzero(im)] # Ignore the background im = im / (2**16 - 1) # Normalize ax1.hist(im, bins=100) ax1.xaxis.set_major_locator(MultipleLocator(0.4)) ax1.minorticks_on() ax1.set_yticks([]) ax1.set_xlabel('Intensity (a.u.)') ax1.set_ylabel('MRI density') # Load the EEG data n_samples, n_rows = 800, 4 with cbook.get_sample_data('eeg.dat') as eegfile: data = np.fromfile(eegfile, dtype=float).reshape((n_samples, n_rows)) t = 10 * np.arange(n_samples) / n_samples # Plot the EEG ticklocs = [] ax2 = fig.add_subplot(2, 1, 2) ax2.set_xlim(0, 10) ax2.set_xticks(np.arange(10)) dmin = data.min() dmax = data.max() dr = (dmax - dmin) * 0.7 # Crowd them a bit. y0 = dmin y1 = (n_rows - 1) * dr + dmax ax2.set_ylim(y0, y1) segs = [] for i in range(n_rows): segs.append(np.column_stack((t, data[:, i]))) ticklocs.append(i * dr) offsets = np.zeros((n_rows, 2), dtype=float) offsets[:, 1] = ticklocs lines = LineCollection(segs, offsets=offsets, transOffset=None) ax2.add_collection(lines) # Set the yticks to use axes coordinates on the y axis ax2.set_yticks(ticklocs) ax2.set_yticklabels(['PG3', 'PG5', 'PG7', 'PG9']) ax2.set_xlabel('Time (s)') plt.tight_layout() plt.show()
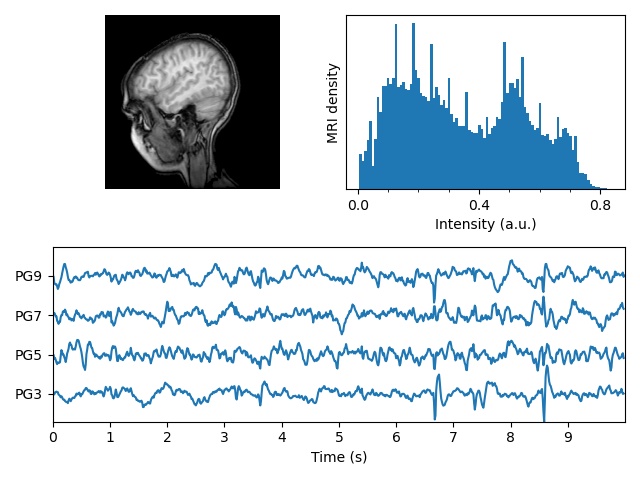
""" =============== Watermark image =============== Using a PNG file as a watermark. """ import numpy as np import matplotlib.cbook as cbook import matplotlib.image as image import matplotlib.pyplot as plt with cbook.get_sample_data('logo2.png') as file: im = image.imread(file) fig, ax = plt.subplots() ax.plot(np.sin(10 * np.linspace(0, 1)), '-o', ms=20, alpha=0.7, mfc='orange') ax.grid() fig.figimage(im, 10, 10, zorder=3, alpha=.5) plt.savefig('watermark.jpg') plt.show() ############################################################################# # # ------------ # # References # """""""""" # # The use of the following functions, methods, classes and modules is shown # in this example: import matplotlib matplotlib.image matplotlib.image.imread matplotlib.pyplot.imread matplotlib.figure.Figure.figimage
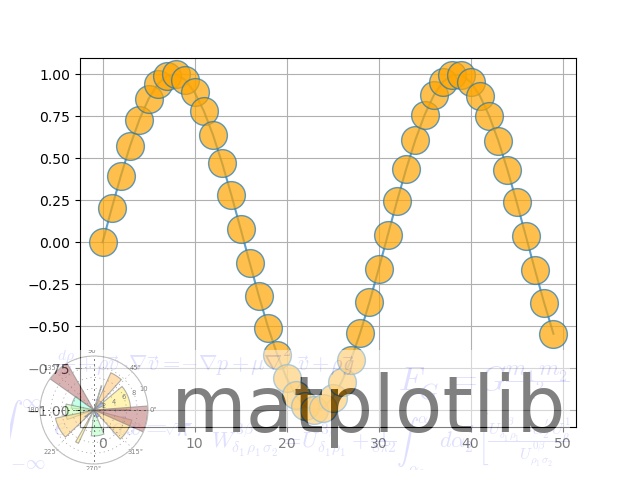
""" ====================== Whats New 0.99 Mplot3d ====================== Create a 3D surface plot. """ import numpy as np import matplotlib.pyplot as plt from matplotlib import cm from mpl_toolkits.mplot3d import Axes3D X = np.arange(-5, 5, 0.25) Y = np.arange(-5, 5, 0.25) X, Y = np.meshgrid(X, Y) R = np.sqrt(X**2 + Y**2) Z = np.sin(R) fig = plt.figure() ax = Axes3D(fig) ax.plot_surface(X, Y, Z, rstride=1, cstride=1, cmap=cm.viridis) plt.savefig('Axes3D.jpg') plt.show() ############################################################################# # # ------------ # # References # """""""""" # # The use of the following functions, methods, classes and modules is shown # in this example: import mpl_toolkits mpl_toolkits.mplot3d.Axes3D mpl_toolkits.mplot3d.Axes3D.plot_surface

Nice read, I just passed this onto a friend who was doing
some research on that. And he just bought me lunch since I found it for him smile So let me rephrase that: Thank you for lunch!
Also visit my blog :: Pellamore Review
Howdy! I simply wish to give you a big thumbs up for the excellent information you
have got here on this post. I am returning to your blog for more soon.
This is the right webpage for anybody who wants to find out about this
topic. You realize a whole lot its almost hard to argue with you (not that I actually would want to…HaHa).
You definitely put a fresh spin on a subject which has been written about for many years.
Great stuff, just wonderful!
my web page; SynerSooth CBD Reviews
Excellent story it is definitely. I’ve been looking for
this info.
Feel free to surf to my blog post … Keto Premium One Shot
Very interesting subject, thank you for putting up.
Also visit my blog – Cryogen Portable AC
Keep functioning ,splendid job!
Also visit my web page … Nature Fused Anti Aging Cream
I was just searching for this information for some time.
After 6 hours of continuous Googleing, at last I got it in your site.
I wonder what is the lack of Google strategy that don’t rank this kind of informative websites in top of the list.
Usually the top web sites are full of garbage.
Feel free to surf to my blog … Zenzi CBD Gummies