Step 1. remove old version and install latest Docker
sudo yum remove docker docker-common docker-selinux docker-engine
sudo yum-config-manager –add-repo https://download.docker.com/linux/centos/docker-ce.repo
sudo yum install docker-ce
If you got: Docker Error – Requires: container-selinux >= 2:2.74
Solution:
Go to http://mirror.centos.org/centos/7/extras/x86_64/Packages/
Find the latest version for container-selinux i.e. container-selinux-2.21-1.el7.noarch.rpm
Run the following command on your terminal: $
sudo yum install -y yum install http://mirror.centos.org/centos/7/extras/x86_64/Packages/container-selinux-2.107-1.el7_6.noarch.rpm
yum install -y yum install http://mirror.centos.org/centos/7/extras/x86_64/Packages/fuse3-libs-3.6.1-4.el7.x86_64.rpm
yum install -y yum install http://mirror.centos.org/centos/7/extras/x86_64/Packages/fuse-overlayfs-0.7.2-6.el7_8.x86_64.rpm
Step 2. enable docker service and start it
$ sudo systemctl enable docker.service
$ sudo systemctl start docker.service ## <-- Start docker ##
$ sudo systemctl stop docker.service ## <-- Stop docker ##
$ sudo systemctl restart docker.service ## <-- Restart docker ##
$ sudo systemctl status docker.service ## <-- Get status of docker ##
$ ip a list docker0
$ docker run hello-world ## <– test your docker installation
Step 3. Get Nagios from https://hub.docker.com/r/jasonrivers/nagios/
docker pull jasonrivers/nagios:latest
docker run –name nagios4 -p 0.0.0.0:8080:80 jasonrivers/nagios:latest
The default credentials for the web interface is nagiosadmin / nagios
alternatively you can use external Nagios configuration & log data with the following:
docker run --name nagios4 \ -v /path-to-nagios/etc/:/opt/nagios/etc/ \ -v /path-to-nagios/var:/opt/nagios/var/ \ -v /path-to-custom-plugins:/opt/Custom-Nagios-Plugins \ -v /path-to-nagiosgraph-var:/opt/nagiosgraph/var \ -v /path-to-nagiosgraph-etc:/opt/nagiosgraph/etc \ -p 0.0.0.0:8080:80 jasonrivers/nagios:latest
You can open it with http://your_server_ip:8080
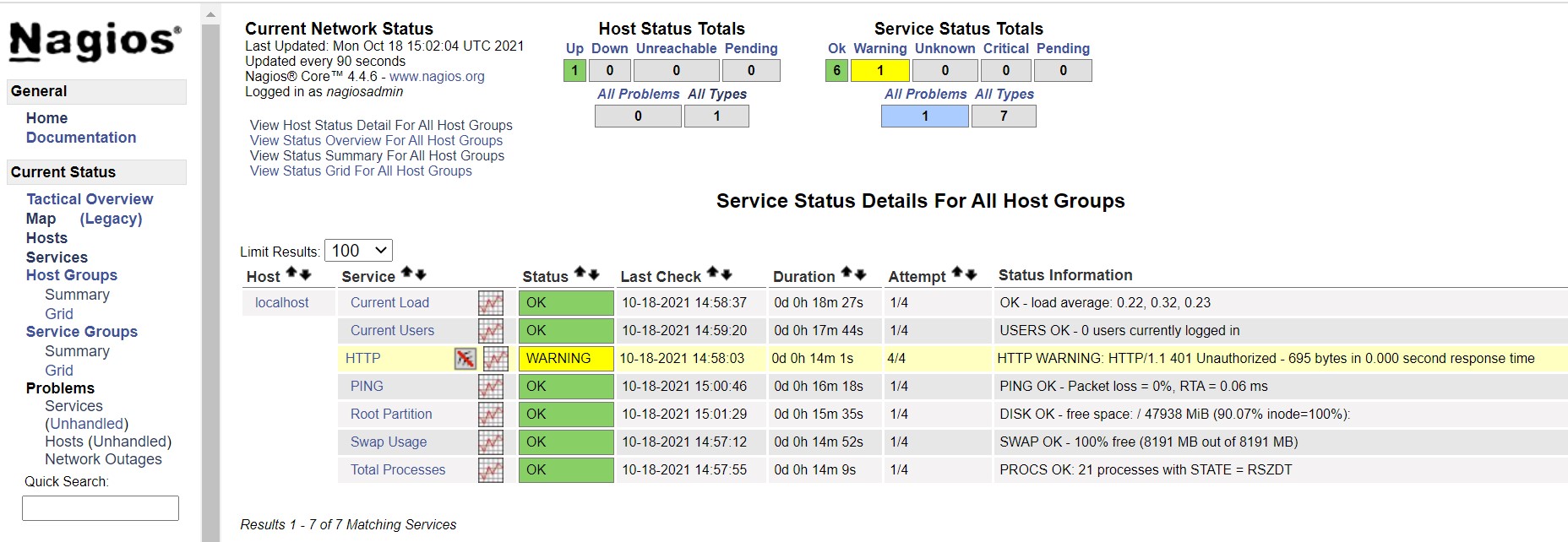
Extra Plugins:
Nagios nrpe [http://exchange.nagios.org/directory/Addons/Monitoring-Agents/NRPE–2D-Nagios-Remote-Plugin-Executor/details]
Nagiosgraph [http://exchange.nagios.org/directory/Addons/Graphing-and-Trending/nagiosgraph/details]
JR-Nagios-Plugins – custom plugins I’ve created [https://github.com/JasonRivers/nagios-plugins]
WL-Nagios-Plugins – custom plugins from William Leibzon [https://github.com/willixix/WL-NagiosPlugins]
JE-Nagios-Plugins – custom plugins from Justin Ellison [https://github.com/justintime/nagios-plugins]
Step 4. How to edit file and restart nagios inside Docker
docker stop $(docker ps -a -q)
docker rm $(docker ps -a -q)
docker run –name nagios4 -v /root/nagios/etc/:/opt/nagios/etc/ -v /root/nagios/apache2/:/etc/apache2/ -p 0.0.0.0:8080:80 -it -d jasonrivers/nagios:latest
restart nagios inside Docker:
docker exec nagios4 rm etc/service/nagios
docker exec nagios4 ln -s /etc/sv/nagios /etc/service