- Install “Nexus Artifact Uploader” and “Pipeline Utility Steps” Plugins
- Create Valid Jenkins Credentials to Authenticate To Nexus OSS
In this step, we should add a Jenkins Crendential of kind “Username with password” with a valid login to our Nexus instance and let’s give it an Id of “nexus-credentials.”
Go to: http://localhost:8080/credentials/
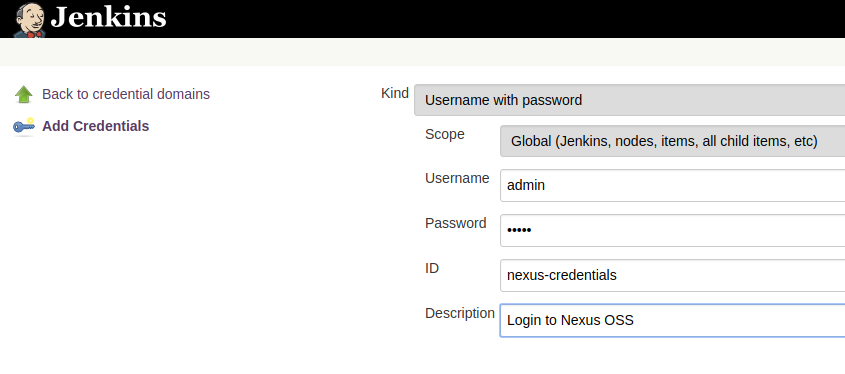
3. Set Up Maven as A Managed Tool, in our case, name is “maven”
4. Publishing Artifacts Using Jenkins Pipelines
pipeline { agent { label "master" } tools { // Note: this should match with the tool name configured in your jenkins instance (JENKINS_URL/configureTools/) maven "maven" } environment { // This can be nexus3 or nexus2 NEXUS_VERSION = "nexus3" // This can be http or https NEXUS_PROTOCOL = "http" // Where your Nexus is running NEXUS_URL = "192.168.0.43:8081" // Repository where we will upload the artifact NEXUS_REPOSITORY = "maven-snapshots" // Jenkins credential id to authenticate to Nexus OSS NEXUS_CREDENTIAL_ID = "nexus-credentials" } stages { stage("clone code") { steps { script { // Let's clone the source git 'https://github.com/zhuby1973/myapp.git'; } } } stage("mvn build") { steps { script { // If you are using Windows then you should use "bat" step // Since unit testing is out of the scope we skip them sh "mvn package -DskipTests=true" } } } stage("publish to nexus") { steps { script { // Read POM xml file using 'readMavenPom' step , this step 'readMavenPom' is included in: https://plugins.jenkins.io/pipeline-utility-steps pom = readMavenPom file: "pom.xml"; // Find built artifact under target folder filesByGlob = findFiles(glob: "target/*.${pom.packaging}"); // Print some info from the artifact found echo "${filesByGlob[0].name} ${filesByGlob[0].path} ${filesByGlob[0].directory} ${filesByGlob[0].length} ${filesByGlob[0].lastModified}" // Extract the path from the File found artifactPath = filesByGlob[0].path; // Assign to a boolean response verifying If the artifact name exists artifactExists = fileExists artifactPath; if(artifactExists) { echo "*** File: ${artifactPath}, group: ${pom.groupId}, packaging: ${pom.packaging}, version ${pom.version}"; nexusArtifactUploader( nexusVersion: NEXUS_VERSION, protocol: NEXUS_PROTOCOL, nexusUrl: NEXUS_URL, groupId: pom.groupId, version: pom.version, repository: NEXUS_REPOSITORY, credentialsId: NEXUS_CREDENTIAL_ID, artifacts: [ // Artifact generated such as .jar, .ear and .war files. [artifactId: pom.artifactId, classifier: '', file: artifactPath, type: pom.packaging], // Lets upload the pom.xml file for additional information for Transitive dependencies [artifactId: pom.artifactId, classifier: '', file: "pom.xml", type: "pom"] ] ); } else { error "*** File: ${artifactPath}, could not be found"; } } } } } }
(base) ubuntu@ubunu2004:/tmp/myapp$ cat pom.xml <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>in.javahome</groupId> <artifactId>myweb</artifactId> <packaging>war</packaging> <version>0.0.9-SNAPSHOT</version> <name>my-app</name> <url>http://maven.apache.org</url> <dependencies> <dependency> <groupId>org.apache.poi</groupId> <artifactId>poi</artifactId> <version>3.7</version> </dependency> <dependency> <groupId>javax.servlet</groupId> <artifactId>javax.servlet-api</artifactId> <version>3.0.1</version> <!-- <scope>provided</scope> --> </dependency> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>3.8.1</version> <scope>test</scope> </dependency> </dependencies> <distributionManagement> <snapshotRepository> <id>nexus</id> <url>http://192.168.0.43:8081/repository/maven-snapshots/</url> </snapshotRepository> <repository> <id>nexus</id> <url>http://192.168.0.43:8081/repository/maven-releases/</url> </repository> </distributionManagement> <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.6.1</version> <configuration> <source>1.7</source> <target>1.7</target> </configuration> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-surefire-plugin</artifactId> <version>2.19</version> </plugin> </plugins> </build> </project>
NOTE: if you failed to upload, then you can try to manually upload, you may got error:
Version policy mismatch, cannot upload SNAPSHOT content to RELEASE repositories…
which means you are trying to upload SNAPSHOT content to RELEASE repositories, you can delete the repositories, and recreate it with Mixed in “Version Policy”
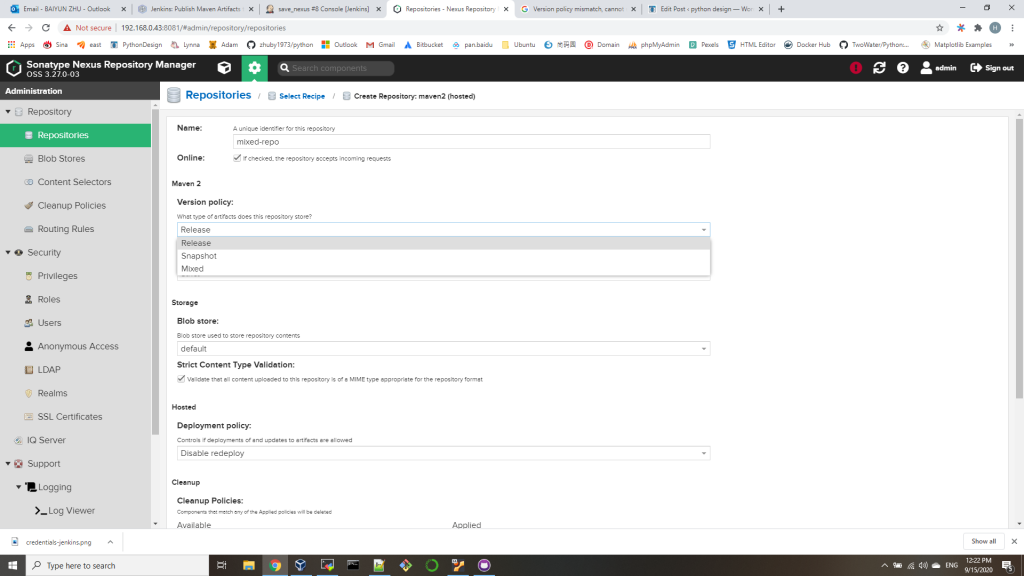