- we can create a simple sample_pipeline job on Jenkins with all default, just one stage in “Pipeline script”
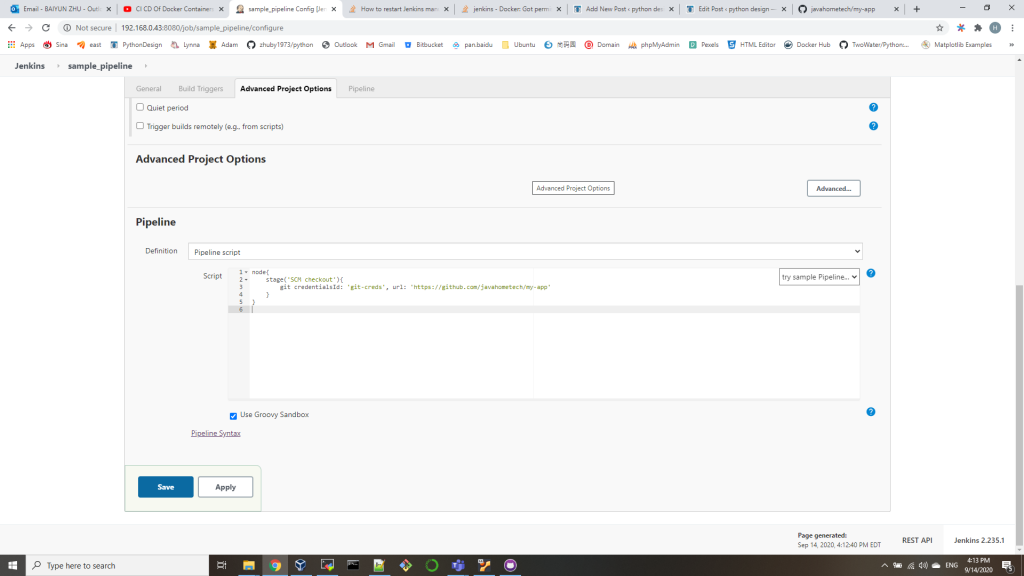
you can setup your GIT account in http://192.168.0.43:8080/job/sample_pipeline/pipeline-syntax/
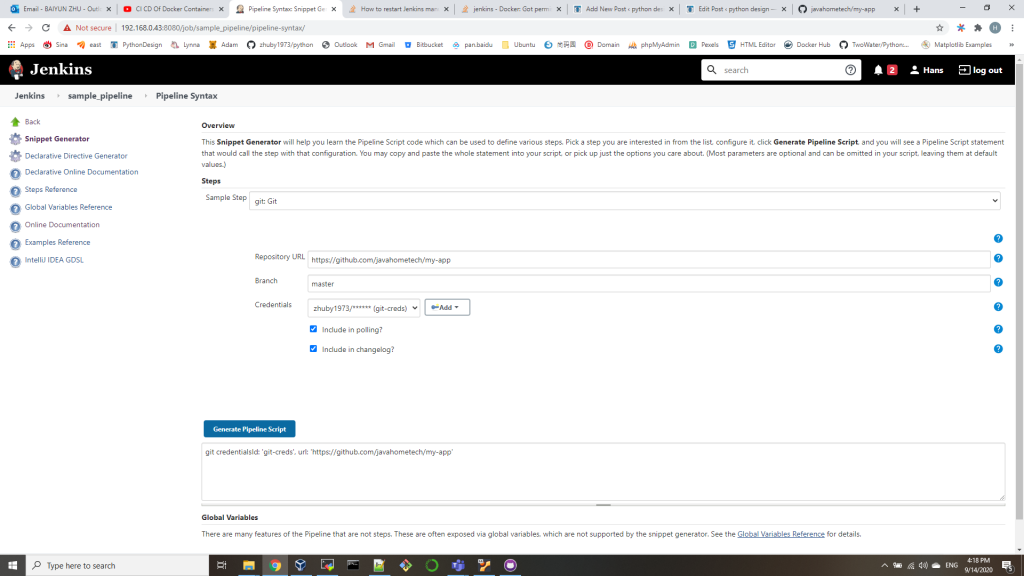
you should be able to run this job without any issue and clone the git repository to your Jenkins VM.
2. we add one more step for mvn Package to generate the war file from cloned source code in /src
node{ stage('SCM checkout'){ git credentialsId: 'git-creds', url: 'https://github.com/javahometech/my-app' } stage('Mvn Package'){ def mvnHome = tool name: 'maven', type: 'maven' def mvnCMD = "${mvnHome}/bin/mvn" sh "${mvnCMD} clean package" } }
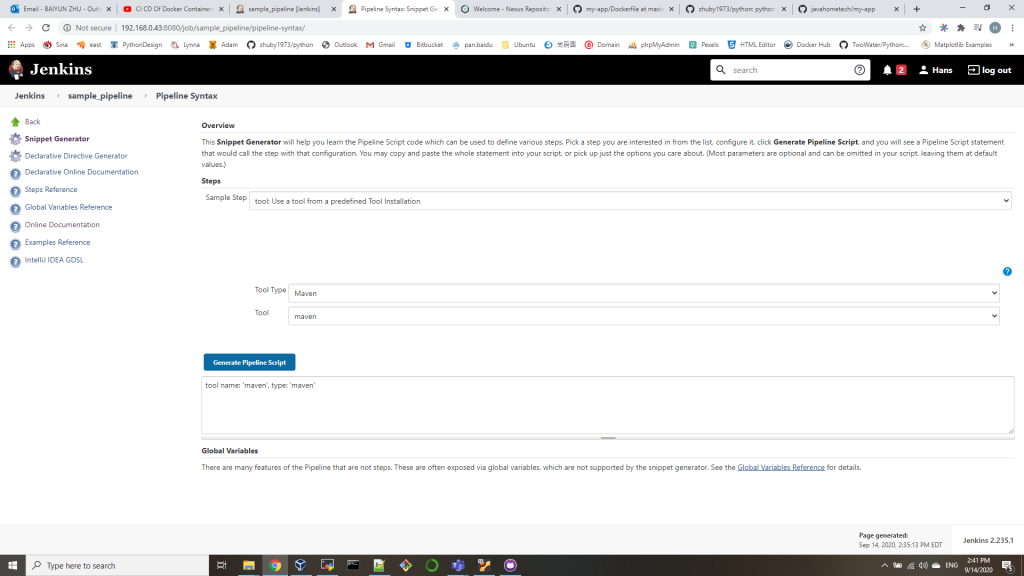
run this job, you will get war generated successfully in ./target
3. add 3rd step to build a Docker image:
node{ stage('SCM checkout'){ git credentialsId: 'git-creds', url: 'https://github.com/javahometech/my-app' } stage('Mvn Package'){ def mvnHome = tool name: 'maven', type: 'maven' def mvnCMD = "${mvnHome}/bin/mvn" sh "${mvnCMD} clean package" } stage('Build Docker Image'){ sh 'docker build -t zhuby1973/myapp:2.0 .' } } (base) ubuntu@ubunu2004:/var/lib/jenkins/workspace/sample_pipeline$ cat Dockerfile FROM tomcat:8 # Take the war and copy to webapps of tomcat COPY target/*.war /usr/local/tomcat/webapps/myweb.war
if you got docker permission error, please fix it with below 3 steps:
1. sudo chmod 664 /var/run/docker.sock 2. sudo usermod -a -G docker jenkins 3. sudo /etc/init.d/jenkins restart
4. push to DockerHub withCredentials
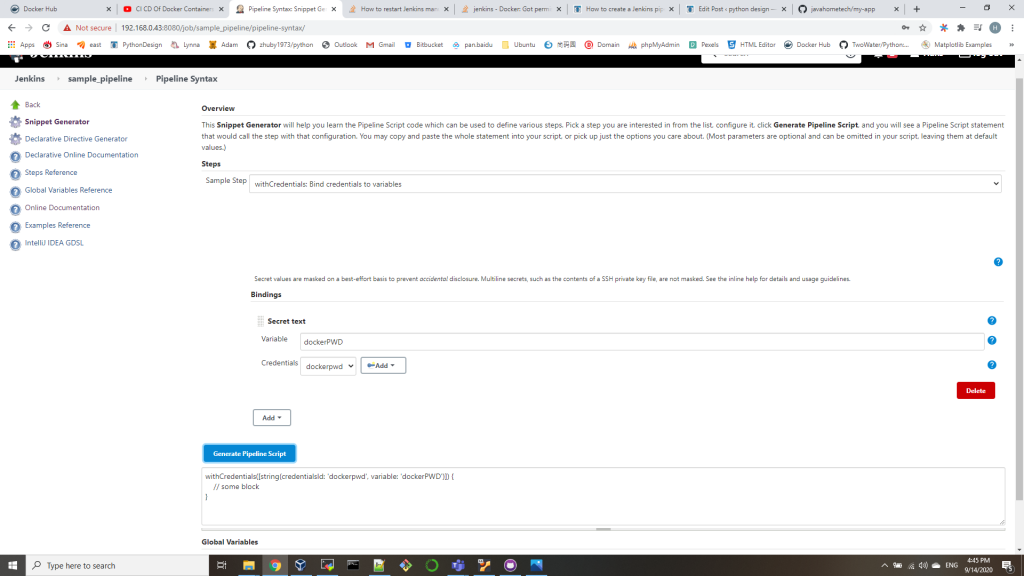
5. deploy the image on DEV server
we will run ssh-keygen on Jenkins VM to get a pair of SSH key, add the public key into DEV server .ssh/authorized_keys, make sure you can logon without credential.
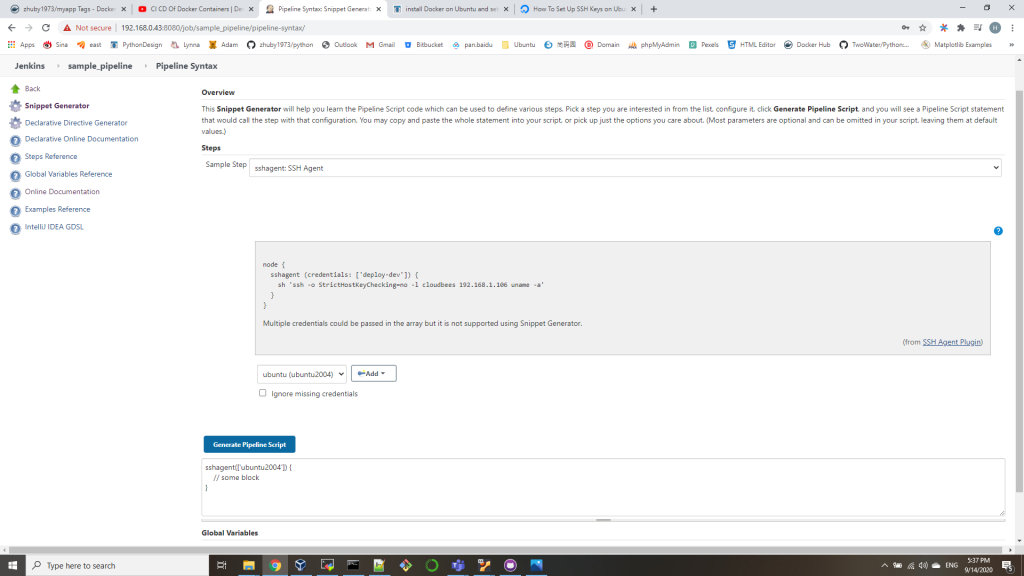
node{ stage('SCM checkout'){ git credentialsId: 'git-creds', url: 'https://github.com/javahometech/my-app' } stage('Mvn Package'){ def mvnHome = tool name: 'maven', type: 'maven' def mvnCMD = "${mvnHome}/bin/mvn" sh "${mvnCMD} clean package" } stage('Build Docker Image'){ sh 'docker build -t zhuby1973/myapp:2.0 .' } stage('Push Docker Image'){ withCredentials([string(credentialsId: 'dockerpwd', variable: 'dockerPWD')]) { sh "docker login -u zhuby1973 -p ${dockerPWD}" } sh 'docker push zhuby1973/myapp:2.0' } stage('Run Container on DEV Server') { def dockerRun = 'sudo docker run -p 8080:8080 -d --name myapp zhuby1973/myapp:2.0' sshagent(['ubuntu2004']) { sh "ssh -o StrictHostKeyChecking=no ubuntu@192.168.0.165 ${dockerRun}" } } }
you can verify the image running on DEV server and also the app url:
http://192.168.0.165:8080/myweb/
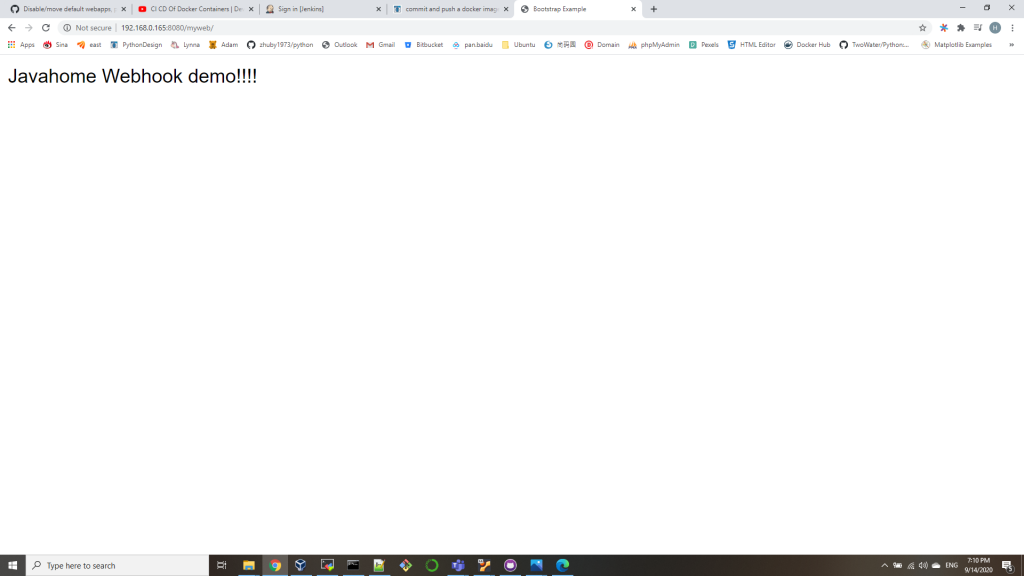
ubuntu@ubuntu2020:~/.ssh$ sudo docker ps
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
f85fe7882cc1 zhuby1973/myapp:2.0 “catalina.sh run” 12 seconds ago Up 9 seconds 0.0.0.0:8080->8080/tcp myapp