- install opencv
pip install opencv-python - Face detection using Haar cascades is a machine learning based approach where a cascade function is trained with a set of input data. OpenCV already contains many pre-trained classifiers for face, eyes, smiles, etc.. Today we will be using the face classifier. You can experiment with other classifiers as well.
You need to download the trained classifier XML file (haarcascade_frontalface_default.xml), which is available in OpenCv’s GitHub repository. Save it to your working location. - download logo.jpg from internet
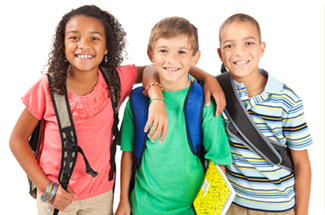
4. python3 code to detect faces in image
import cv2 # Load the cascade #face_cascade = cv2.CascadeClassifier('haarcascade_frontalface_default.xml') face_cascade = cv2.CascadeClassifier(cv2.data.haarcascades + "haarcascade_frontalface_default.xml") # Read the input image img = cv2.imread('logo.jpg') # Convert into grayscale gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) # Detect faces faces = face_cascade.detectMultiScale(gray, 1.1, 4) # Draw rectangle around the faces for (x, y, w, h) in faces: cv2.rectangle(img, (x, y), (x+w, y+h), (255, 0, 0), 2) crop_face = img[y:y + h, x:x + w] # save faces cv2.imwrite(str(x) + '_' + str(y) + '_face.jpg', crop_face) # Display the output cv2.imshow('img', img) cv2.imshow("imgcropped", crop_face) cv2.waitKey()
5. run it with pythoh cv2_sample.py, you will find faces detected and cropped faces saved:
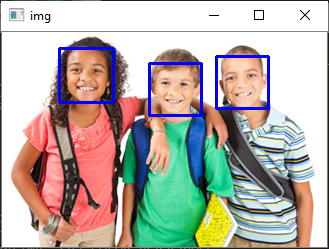
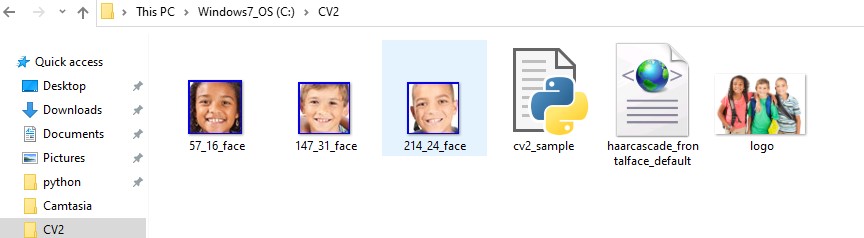